Assignments > P1: Make an Interactive Animation
Due on Thu, 05/21 @ 11:59PM. 40 Points.
This assignment asks you to apply what you’ve learned to create an animated, interactive terrarium or aquarium. To get an idea of what you might make, please see the sample videos (as well as the animated gif below). Many (in fact most) of these samples have gone above and beyond the assignment specifications (and thus earned extra credit), but please know that meeting the assignment’s minimum requirements is sufficient for earning all 40 points. Please calibrate your implementation according to your experience level with programming (i.e. do more if you’re more experienced): you’ll get out of it what you put into it. See the rubric below to get a sense of how you will be evaluated on this assignment. Then, download the starter files (above).
Created by Rachel Smith (Berkeley City College)
Part 1: Create a make_creature function (7 points)
In helpers.py
, create a custom function called make_creature
that makes a creature of your choosing. Feel free to use/modify/improve upon the function you already made in HW3. It should have at least 4 parameters that enable the calling function to specify arguments for:
- canvas (positional): a reference to the canvas object.
- center (positional): a tuple representing the (x, y) coordinate of the centerpoint of the creature.
- size (keyword): an integer that controls the size of the creature.
- tag (keyword): a string that gives the creature a unique tag name.
Important: in order for the shapes that constitute your creature to “belong” together, each shape has to be tagged. Please see the sample
make_creature
function in helpers.py to see how tagging works.
You may also add any additional keyword parameters (i.e., they must be optional, not required) of your choosing to make your creature even more customizable (e.g.: primary_color=’red’, has_sunglasses=True, etc.). Or, feel free to use the random module to create creatures with ‘surprise’ features.
You are also welcome to create additional creature functions (if you want different kinds of creatures to be present in your terrarium / aquarium). That said, please ensure that your main creature function is called make_creature
(for our grading pipeline). Any additional creature functions can be named anything that you want and work in any way you want. Up to 2 points will be given for fantastic beasts.
Part 2: Create a make_landscape_object function (7 points)
You have already experimented with stars and bubbles in Homework 4. Here, you will make an object of your own choosing (e.g. a tree, a building, a rock, grass, coral, etc.). To do this, you will create a custom function called make_landscape_object
, in helpers.py, that draws a landscape feature of your choosing. It should have at least 3 parameters that enable the calling function to specify arguments for:
- canvas (positional): a reference to the canvas object.
- center (positional): a tuple representing the (x, y) coordinate of the centerpoint of the background object.
- size (keyword): an integer that controls the width of the background object.
- tag (keyword): a string that gives the landscape object a unique tag name.
You may also add any additional keyword parameters of your choosing to make your landscape object even more customizable (e.g.: color=’red’, texture=’rough’).
You are also welcome to create several different landscape feature functions to make different landscape features. Note that while your main landscape function must be called make_landscape_object
, any additional landscape feature functions can be named anything that you want. Up to 2 points will be given for fantastic landscape features.
3. Render your aquarium / terrarium (7 points)
In main.py
, you will render your landscape. This involves (a) instantiating some background creatures (using your make_creature function), and (b) creating some landscape features (using your make_landscape_object function).
a. Background creatures
Instantiate at least 5 different “background” creatures in your landscape, located at different positions, and with varying sizes (and colors and features — optional). You may use a loop (and perhaps a random function) to position your background creatures, or you can intentionally place your background creatures in specific places — or some combination of the two.
b. Landscape features
Instantiate at least 5 instances of your landscape feature (in the same manner as you did with your background creatures).
Part 4. Animate your landscape (15 points)
Animate the creatures in your landscape by picking five of the following effects to implement:
Points | Task | |
---|---|---|
1 | 3 points | Animate your creatures (and your environmental features if it makes sense to do so). If creatures / environmental features move off of the screen, recreate them on the other side or have them bounce off the side. |
2 | 3 points | Experiment with different kinds of motion. Instead of your creatures moving linearly at a constant speed, you can experiment with the math.sin and math.cos functions (or any others techniques) to make your creature oscillate, accelerate, decelerate, etc. |
3 | 3 points | Spawn a new creature when the user clicks the screen. |
4 | 3 points | Animate each of your creatures so that their movement is slightly different (different speeds, different movement patterns). |
5 | 3 points | Enable your user to control one or more of your creatures using keyboard events (pressing the up/down arrow, using the spacebar, etc.) |
6 | 3 points | Periodically add or remove creatures to/from your scene. |
7 | 3 points | When you click a creature, remove it from the screen |
8 | 3 points | Detect creature collisions, and do something interesting if creatures collide |
9 | 3 points | Enable the user to reposition a creature or landscape feature by dragging it |
10 | 3 points | Create some game mechanics (space bar jumps or shoots, drag ‘flings’ an object, etc.). |
11 | 3 points | Make your creature or landscape feature periodically change colors. |
NOTE: If you surpass the 15 points (i.e. 5 features), we will award up to 6 points extra credit for pursuing additional enhancements from the list above. So, a sixth feature would be 3 points extra credit, for example.
Part 5. Create a short video (1 point)
Please create a short (like ~15 seconds) video of your terrarium / aquarium in action. To do this:
- Take a screencast. For Mac users,QuickTime works, and is free. Zoom also enables you to record your screen.
- Upload it to Google Drive.
- Share your video so that anyone with link can view.
Reference Materials
Code Samples
I have created some sample code (in demos
folder) to help you to understand how to interact with canvas using the keyboard and mouse. Check out the sample code to get some implementation ideas.
TKinter Documentation
You will also want to refer to the tkinter documentation. Here are some useful sites (but feel free to do your own research):
Old Documentation
From Python 2.x (still quite useful):
- tkinter events: http://effbot.org/tkinterbook/tkinter-events-and-bindings.htm
- tkinter canvas: http://effbot.org/tkinterbook/canvas.htm
Newer Tutorials (Python 3.x)
- https://www.tutorialspoint.com/python3/python_gui_programming.htm
- https://dzone.com/articles/python-gui-examples-tkinter-tutorial-like-geeks
Policy on sample code and collaboration
YOU MAY….
- Make use of any sample code that I have provided you at any point during the course
- Consult with and incorporate ideas from Internet sources, so long as you are typing the code for yourself (not copying) and understand how every line of it works
- Help each other debug your code and discuss ideas together
YOU MAY NOT…
- Share code — or look at your neighbor’s screen and transcribe their code. We have plagiarism detection software to flag code similarities (e.g. MOSS) — even when whitespace, variable names, and ordering have been changed.
- Blindly copy code from third-party sources and/or incorporate programming techniques that you don’t understand.
- Ask CS majors (or other more experienced people) to write code for you.
What to Turn In
- Your code as a zip file. This should include all of the files that make your code work.
- A link to your video (paste it into the text area on Canvas)
- A (very) short paragraph (bullets fine) indicating which 5 features you implemented for part 4, including any extra credit that you completed. This is critical for helping the TAs look out for the features you implemented. Help us help you get the points you deserve.
Rubric
Feature | Points | Scoring Guidelines |
---|---|---|
Creature function | 7 |
Function enables creature to be customized by size and position.
|
Landscape function | 7 |
Function enables landscape object to be customized by size and position.
|
Render aquarium / terrarium | 7 |
|
Animate your landscape | 15 |
|
Code Quality | 3 |
|
Video | 1 |
|
Extra Credit | Maximum of 6 |
For projects that exceed expectations in any of the following ways:
|
Student Showcase
Check out everyone’s fabulous creations. I have also pasted a few screenshots below to give a little preview of what some of you made. That said, everyone’s landscapes were fantastic, so if yours isn’t featured below, it’s just because I didn’t have time to take a screenshot of everyone’s! Well done.
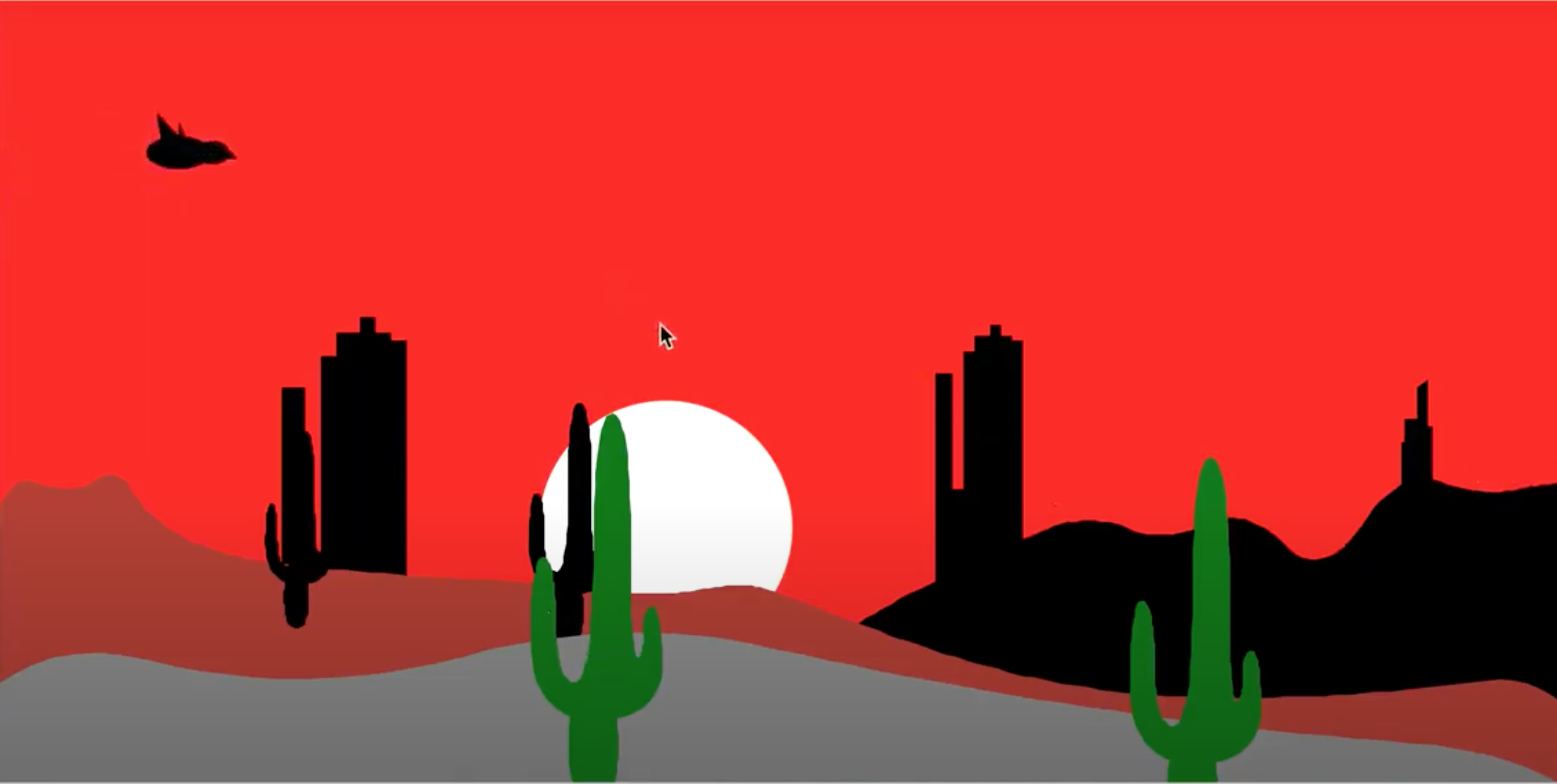
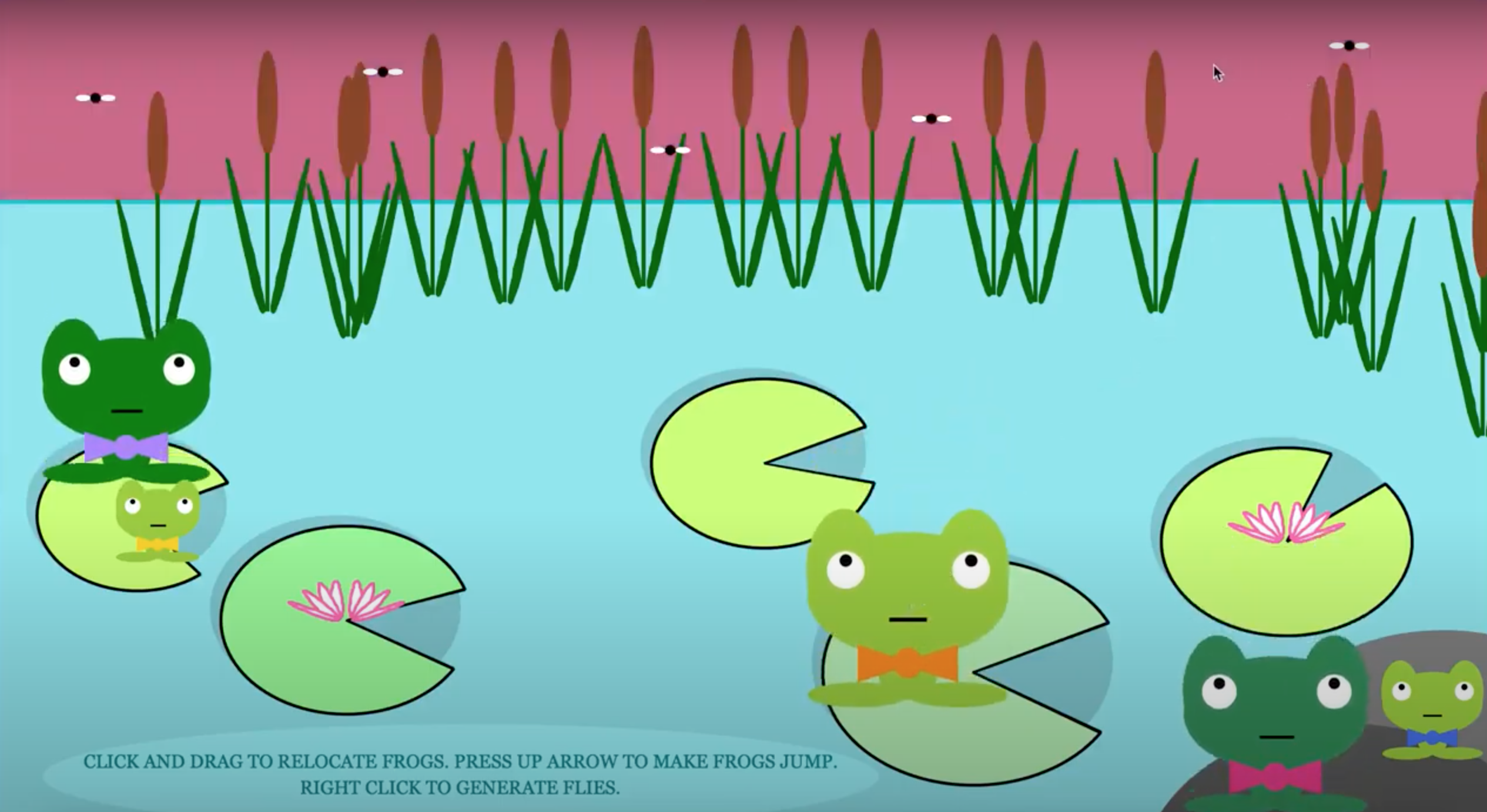
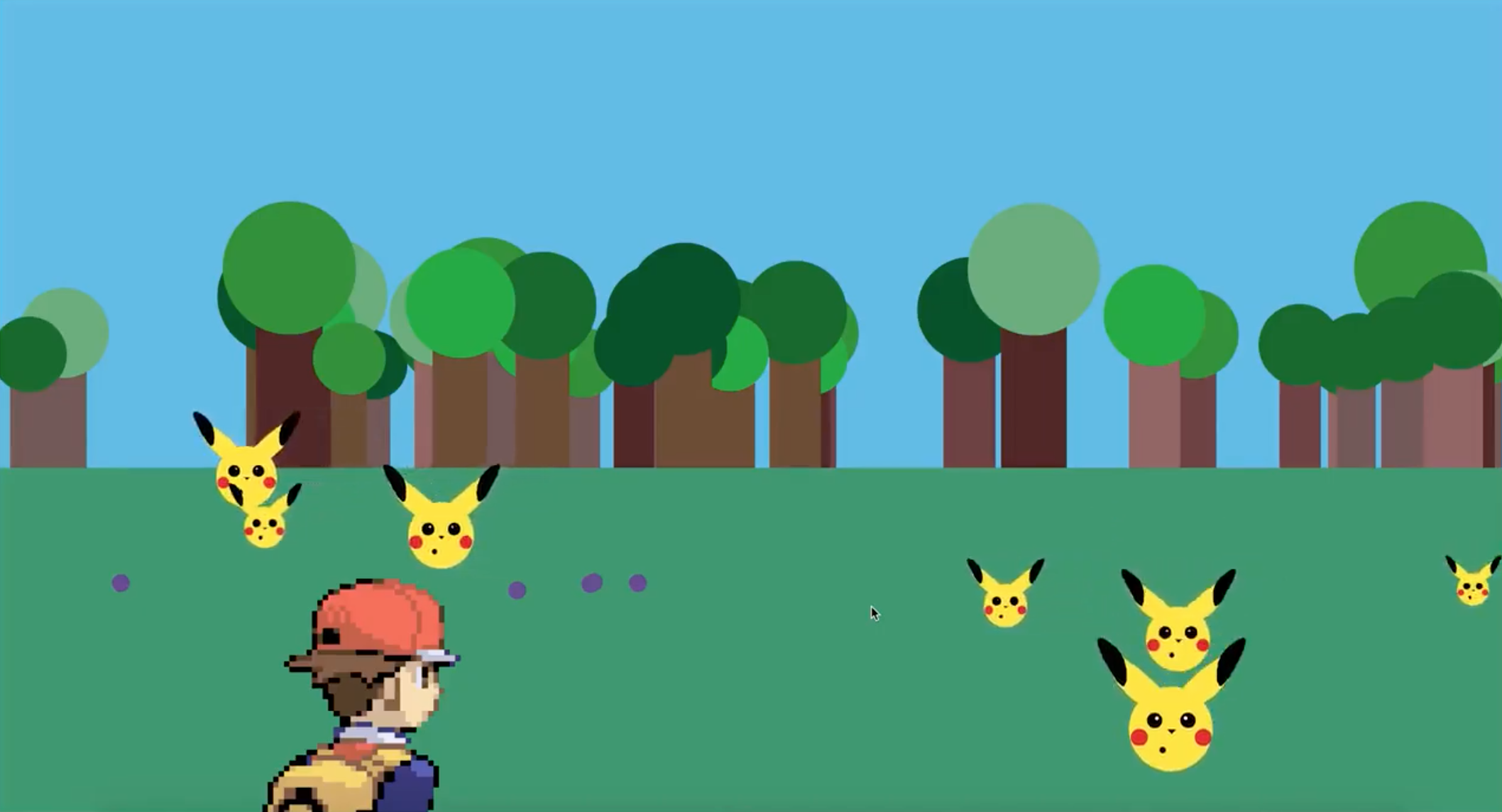
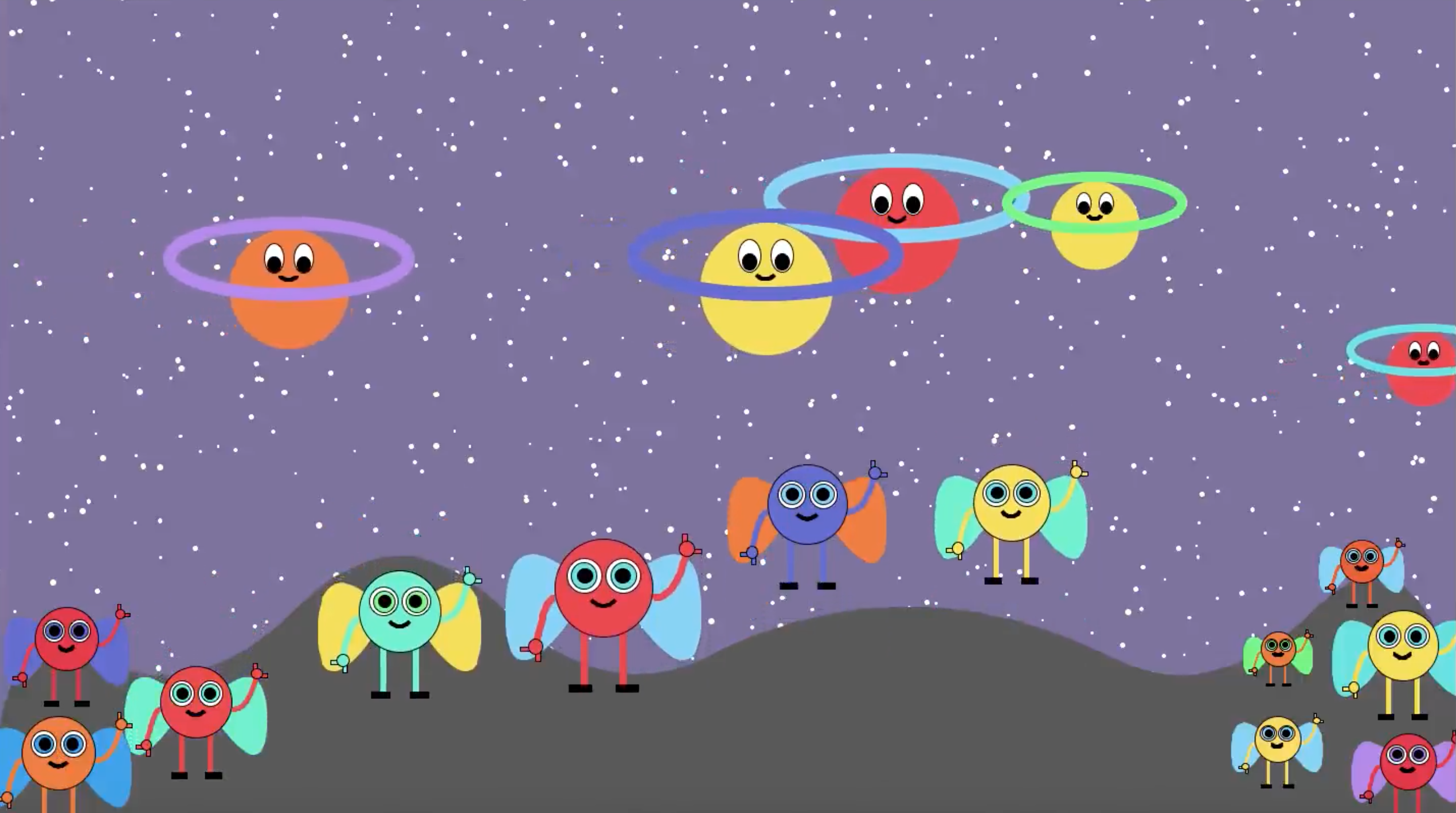
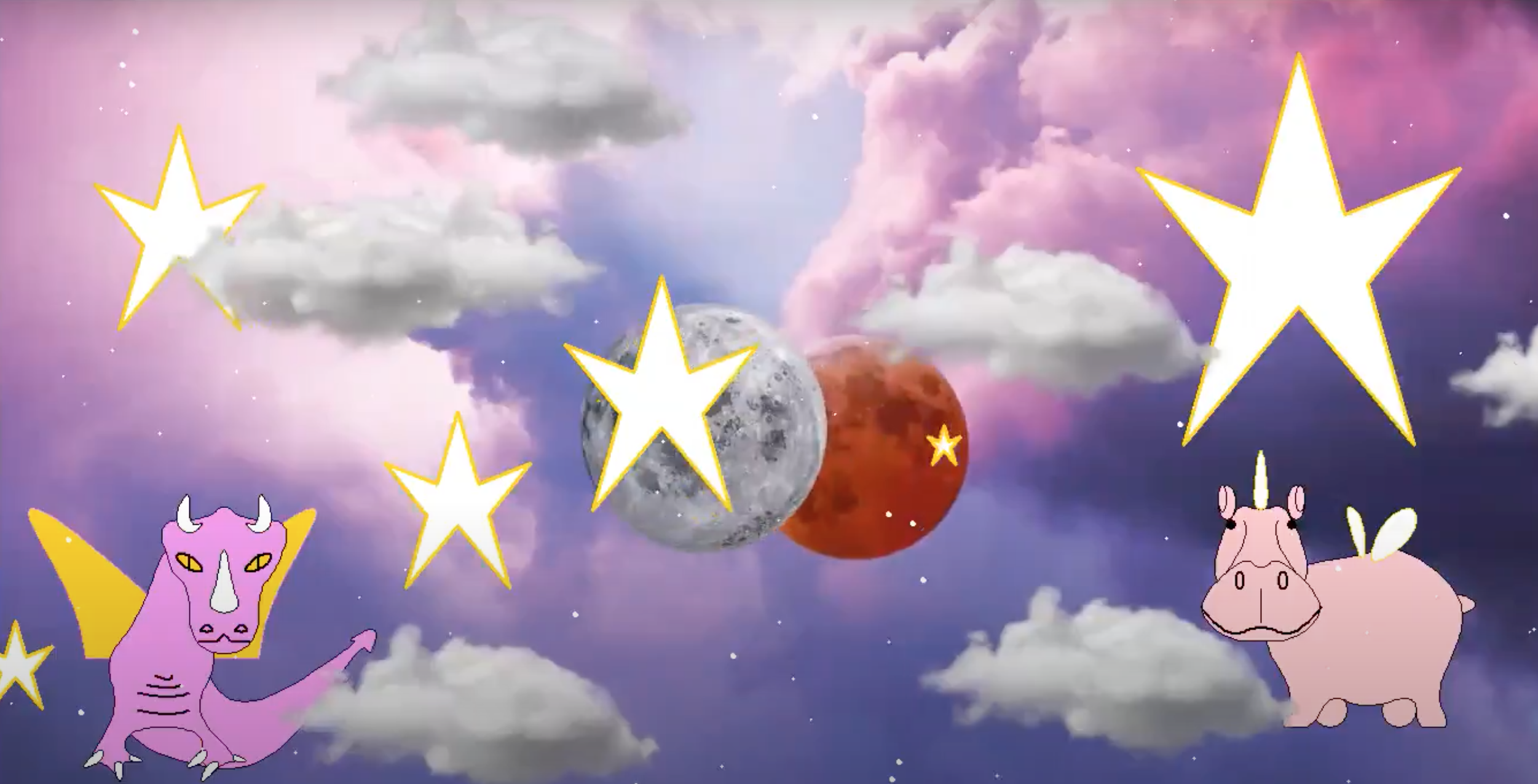
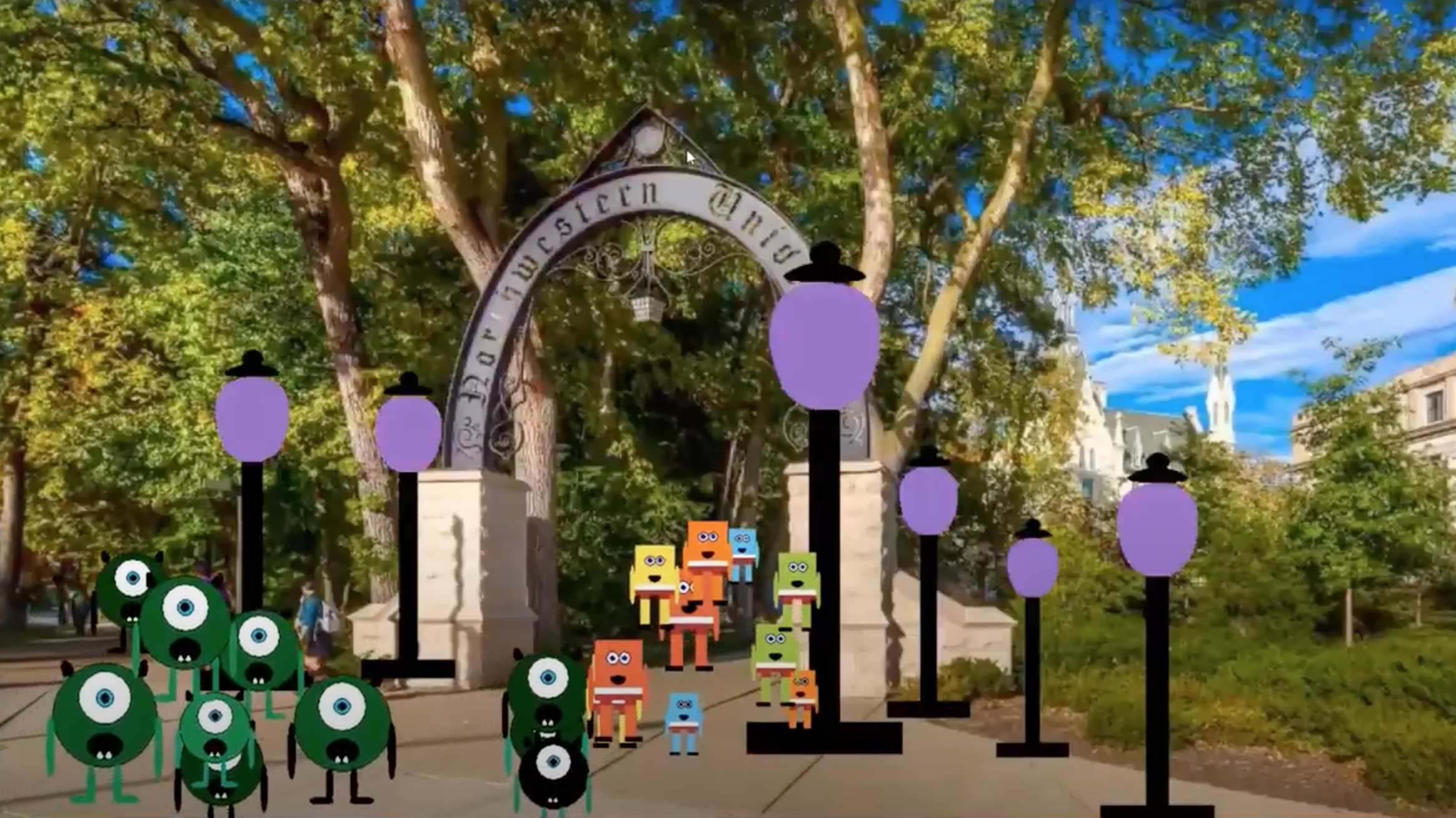
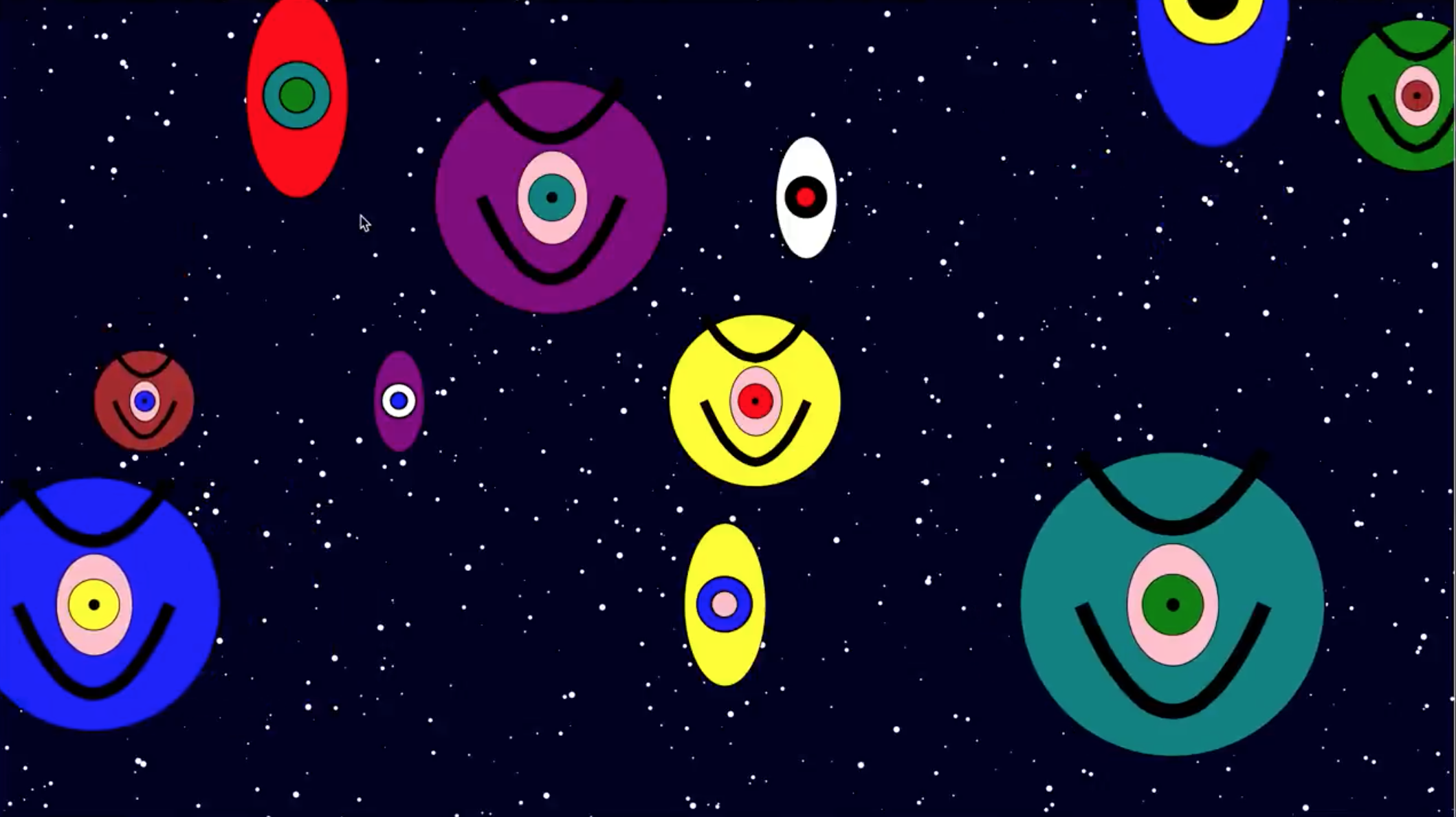
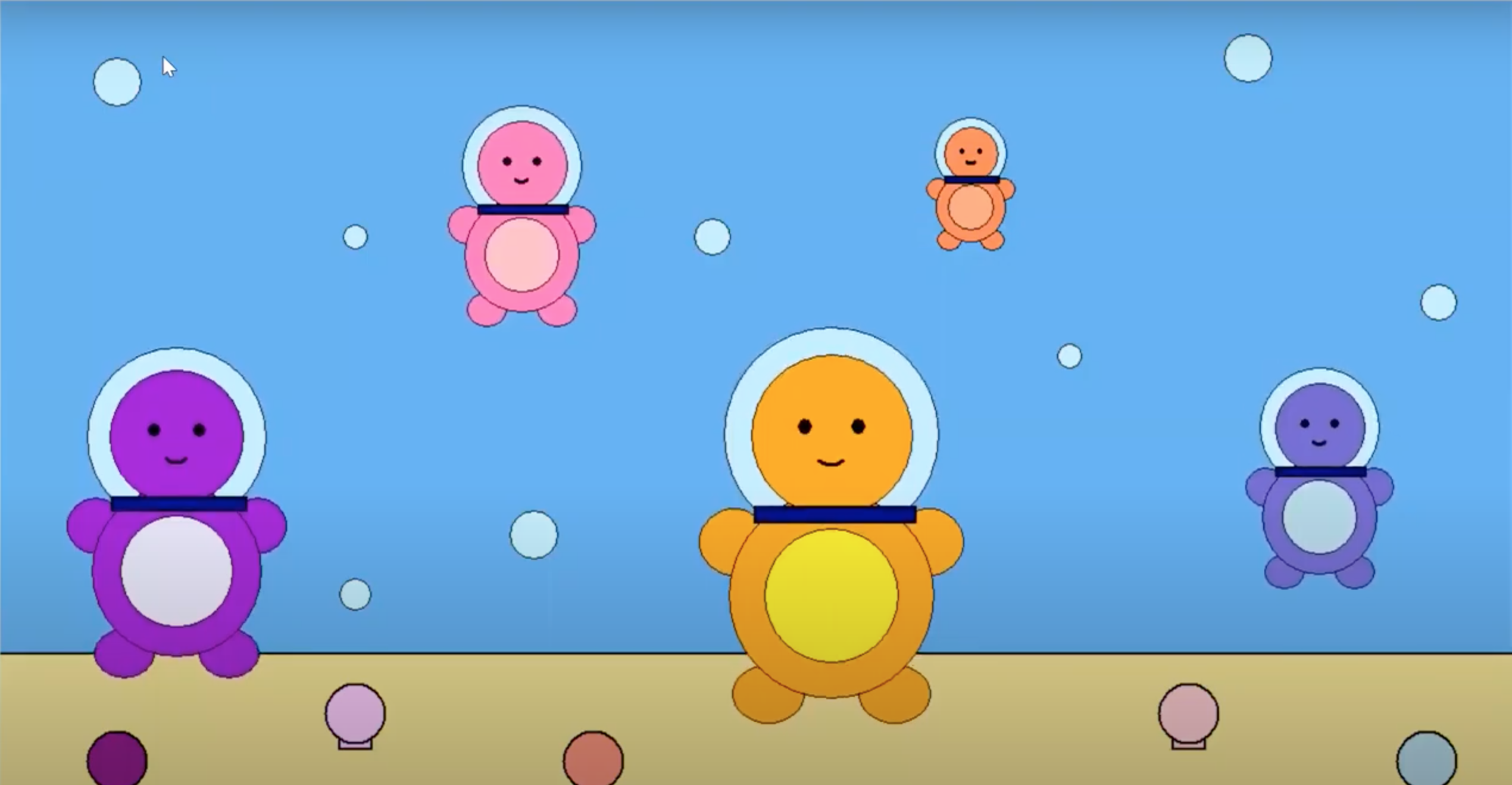
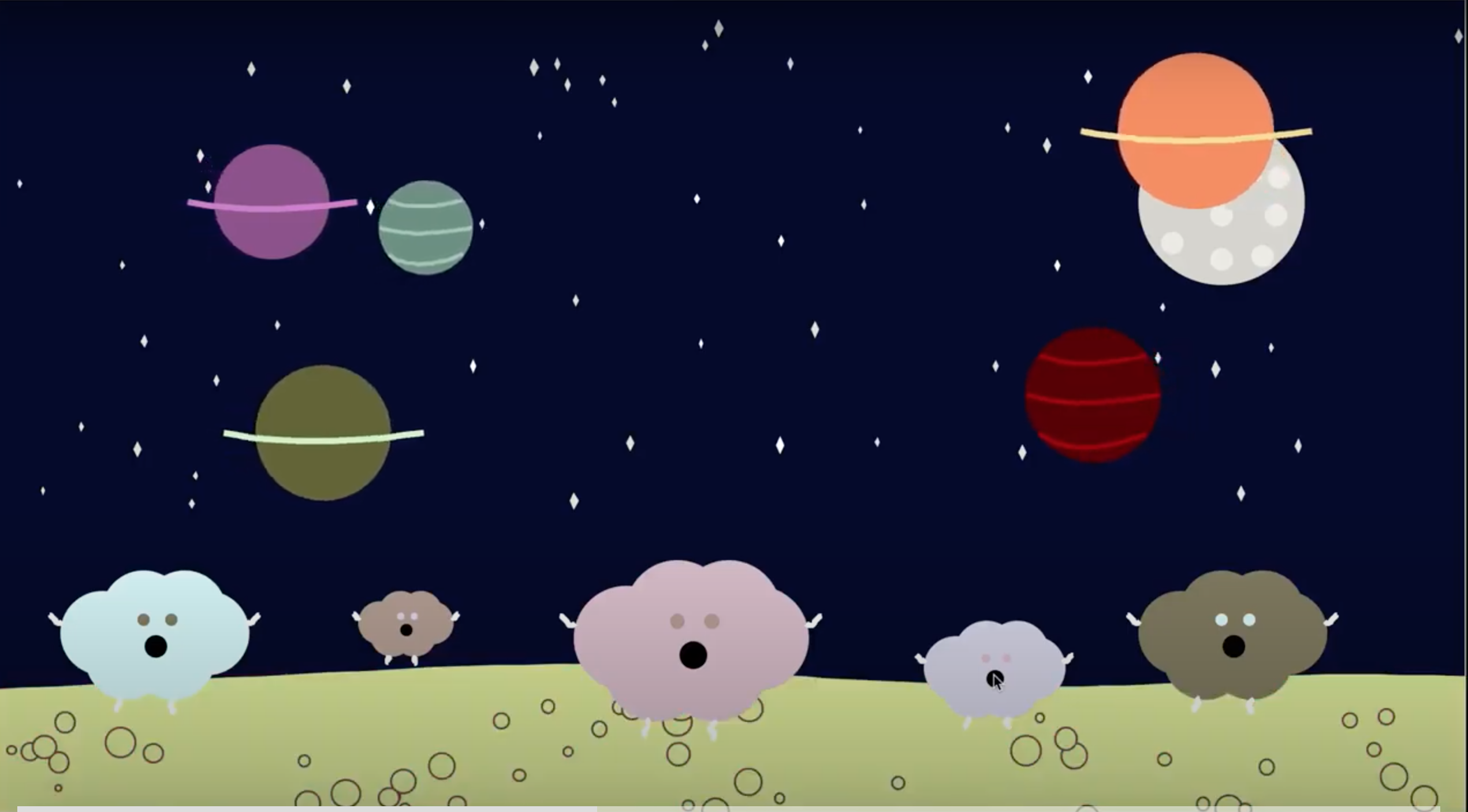
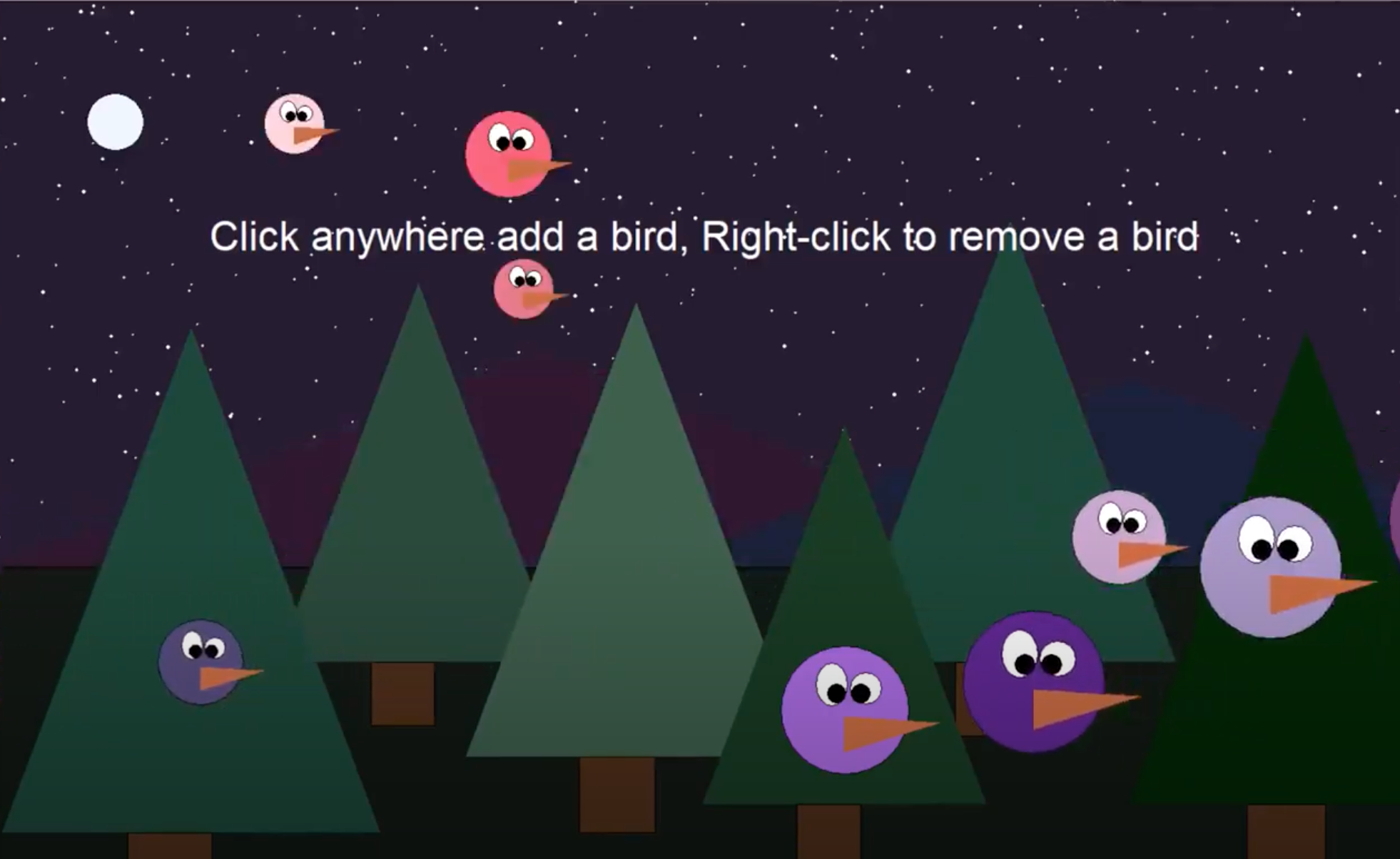
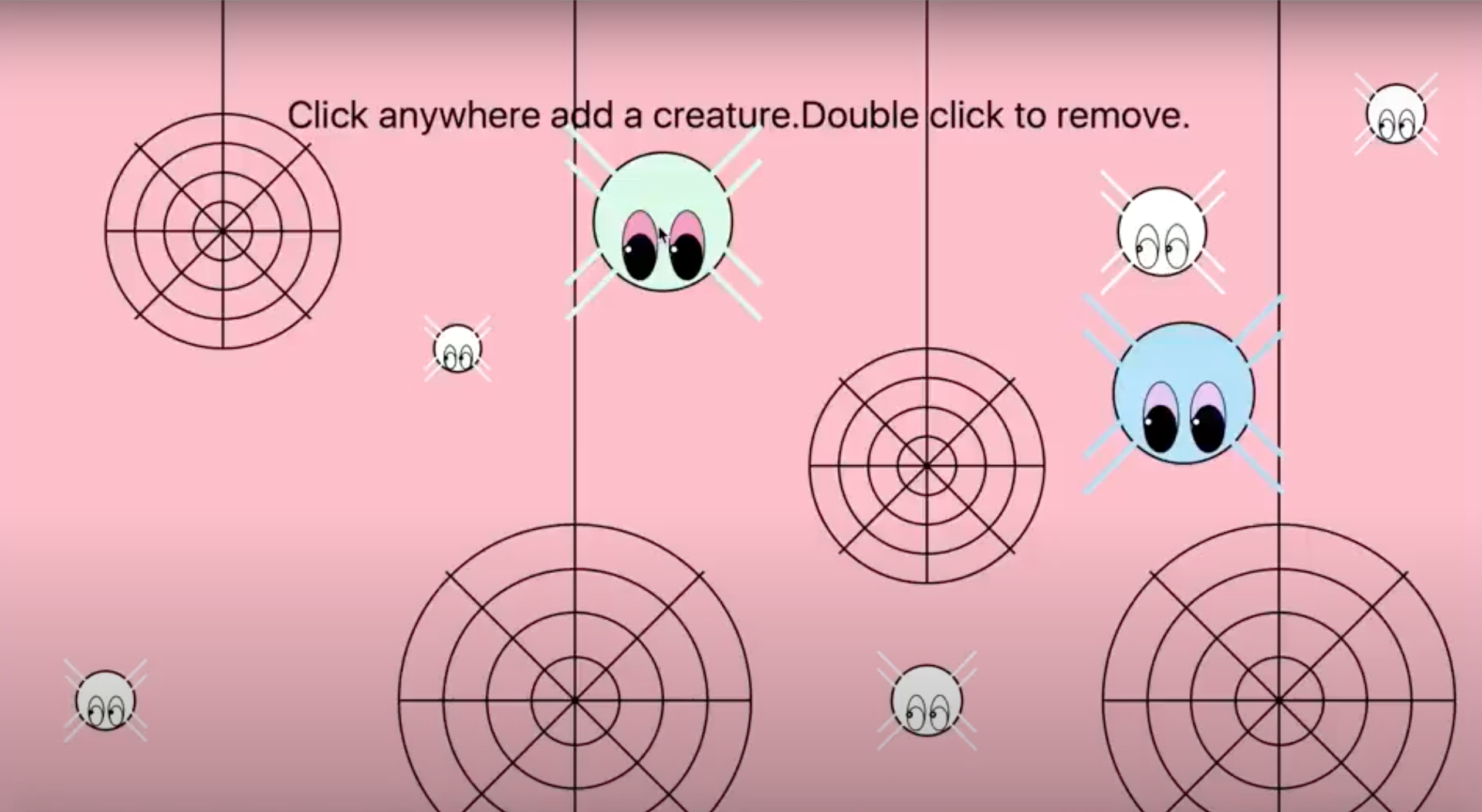
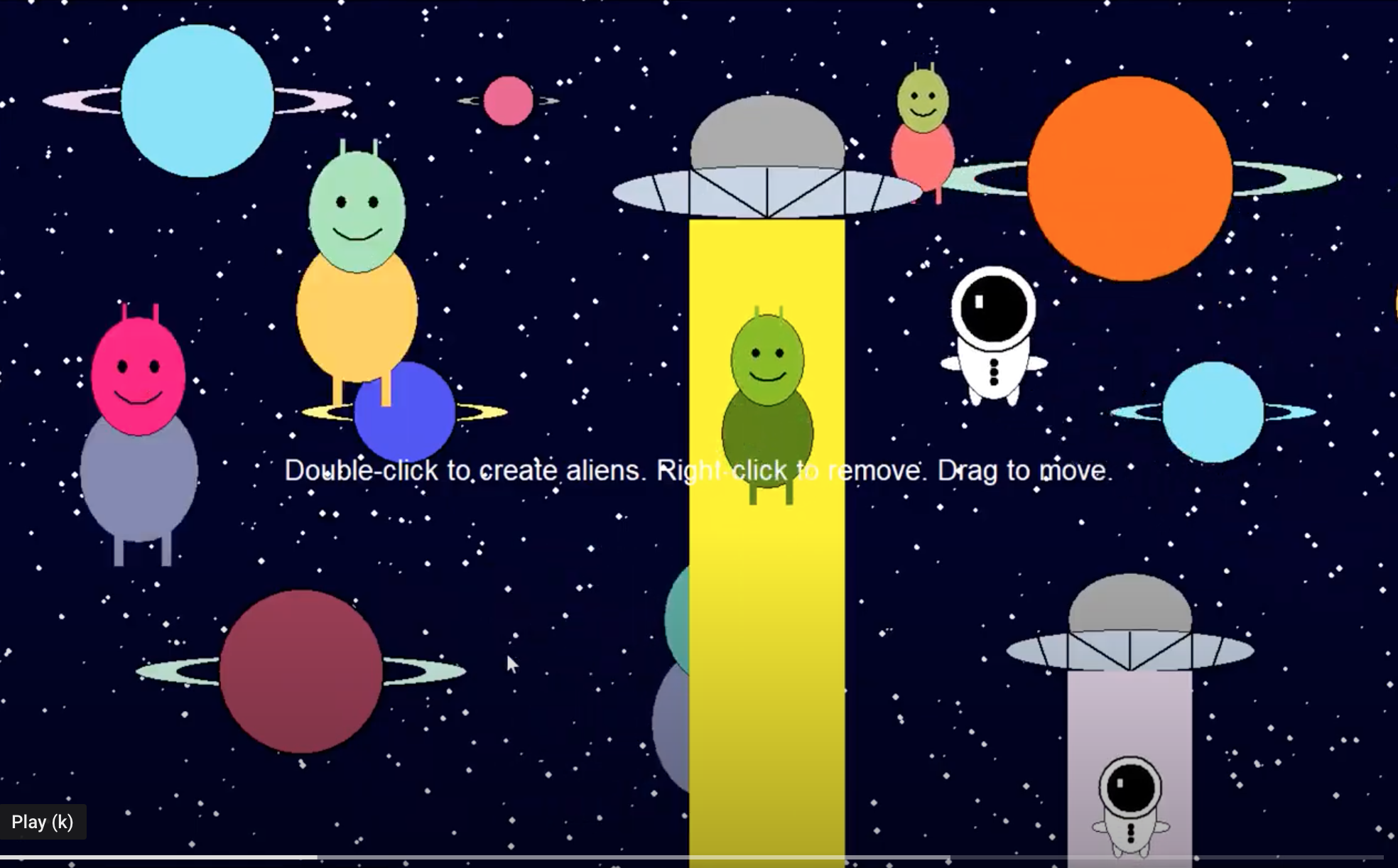
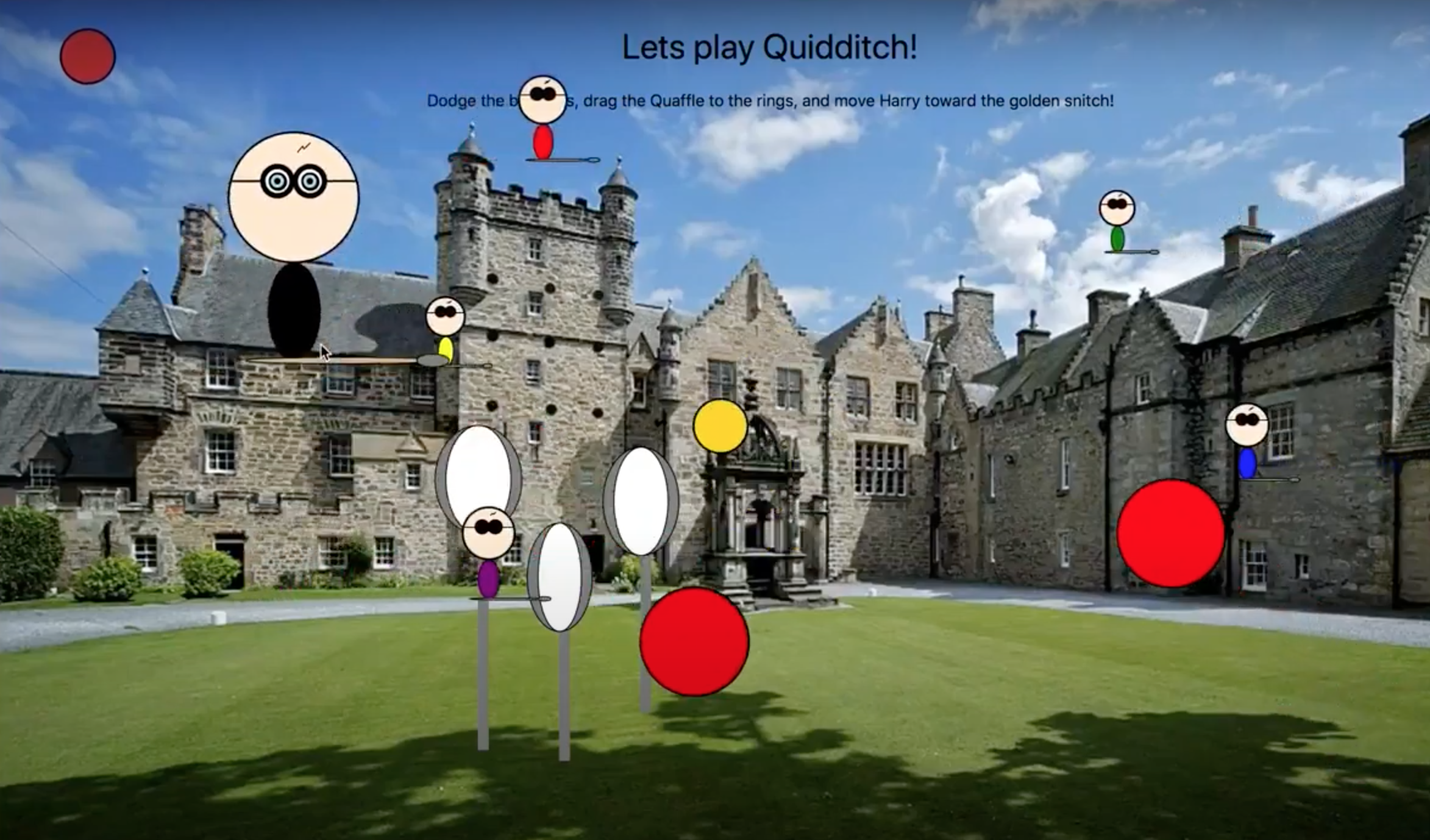